Gradient Descent JavaScript
Gradient Descent is a popular iterative optimization algorithm commonly used in machine learning, specifically in training models such as neural networks. It is an optimization technique that seeks to find the minimum value of a function by iteratively adjusting the parameters. In this article, we will explore how Gradient Descent can be implemented using JavaScript.
Key Takeaways
- Gradient Descent is an iterative optimization algorithm used in machine learning.
- It is used to find the minimum value of a function.
- JavaScript can be used to implement Gradient Descent.
**Gradient Descent** works by **iteratively** adjusting model parameters in the opposite direction of the *gradient* of the loss function with respect to these parameters. This process continues until convergence, i.e., until the algorithm finds the optimal parameters or reaches a predefined stopping condition.
When implementing Gradient Descent in JavaScript, **vectorization** can greatly improve the performance of the algorithm by leveraging the power of linear algebra operations. By performing computations on arrays rather than individual elements, we can take advantage of highly optimized algorithms provided by libraries like **TensorFlow.js**.
Implementing Gradient Descent in JavaScript
The following steps outline a basic implementation of Gradient Descent in JavaScript:
- Initialize the model parameters randomly.
- Calculate the gradient of the loss function with respect to the parameters.
- Update the parameters by subtracting a small learning rate multiplied by the gradient.
- Repeat steps 2 and 3 until convergence.
*When using Gradient Descent, an interesting technique to improve convergence is **learning rate scheduling**, where the learning rate decreases over time. This approach helps the algorithm to take larger steps in the beginning and gradually refine the updates as it gets closer to the minimum.*
Table 1: Comparison of Learning Rates
Learning Rate | Convergence Time |
---|---|
0.1 | 100 iterations |
0.01 | 500 iterations |
0.001 | 1000 iterations |
The **choice of learning rate** is crucial when implementing Gradient Descent. A too small learning rate may lead to slow convergence, while a too large learning rate can cause the algorithm to overshoot the minimum and fail to converge. Therefore, it is important to experiment with different values and observe the convergence behavior.
Improving Gradient Descent with Mini-Batches
In certain scenarios, processing the entire dataset at once can be computationally expensive. An alternative approach is to divide the dataset into smaller **mini-batches** and perform the Gradient Descent updates on each mini-batch. This is known as **mini-batch Gradient Descent**.
Using mini-batches in Gradient Descent can offer several advantages:
- It reduces the memory requirements as only a small batch of data needs to be loaded at a time.
- It introduces noise in the update process, which can help the algorithm to escape local minima and converge to a better solution.
- It can speed up the training process as updating parameters on mini-batches can be more efficient.
Table 2: Comparison of Mini-Batch Sizes
Mini-Batch Size | Convergence Time (Iterations) | Memory Usage |
---|---|---|
10 | 500 iterations | Low |
100 | 300 iterations | Moderate |
1000 | 200 iterations | High |
*Mini-batch size selection should strike a balance between computational efficiency and memory limitations. It is recommended to experiment with different mini-batch sizes to determine the optimal setting for a specific problem.*
Overcoming Local Minima
One limitation of Gradient Descent is its susceptibility to getting stuck in **local minima**, which are suboptimal solutions of the optimization problem. However, there are techniques to overcome this issue:
- Using a **random initialization** of parameters can help to escape some local minima.
- Using **momentum** in the update equation can help the algorithm to continue progressing in the direction of the minimum, even when the gradient is small.
- Employing more advanced optimization algorithms such as **stochastic gradient descent (SGD)** or **Adam** can provide better convergence and handle complex optimization landscapes.
Table 3: Comparison of Optimization Techniques
Technique | Advantages | Disadvantages |
---|---|---|
Gradient Descent | Simple and easy to implement | Slow convergence, susceptible to local minima |
Stochastic Gradient Descent | Faster convergence, handles large datasets well | May converge to suboptimal solutions |
Adam | Efficient, adapts learning rate for each parameter | More complex and requires fine-tuning |
In conclusion, Gradient Descent is a powerful optimization algorithm used to find the minimum value of a function during the training of machine learning models. Implementing Gradient Descent in JavaScript allows for flexibility and ease of use, making it accessible to a wider range of developers. By applying optimization techniques, such as learning rate scheduling, mini-batch processing, and advanced optimization algorithms, it is possible to enhance the performance and convergence of Gradient Descent for different machine learning tasks.
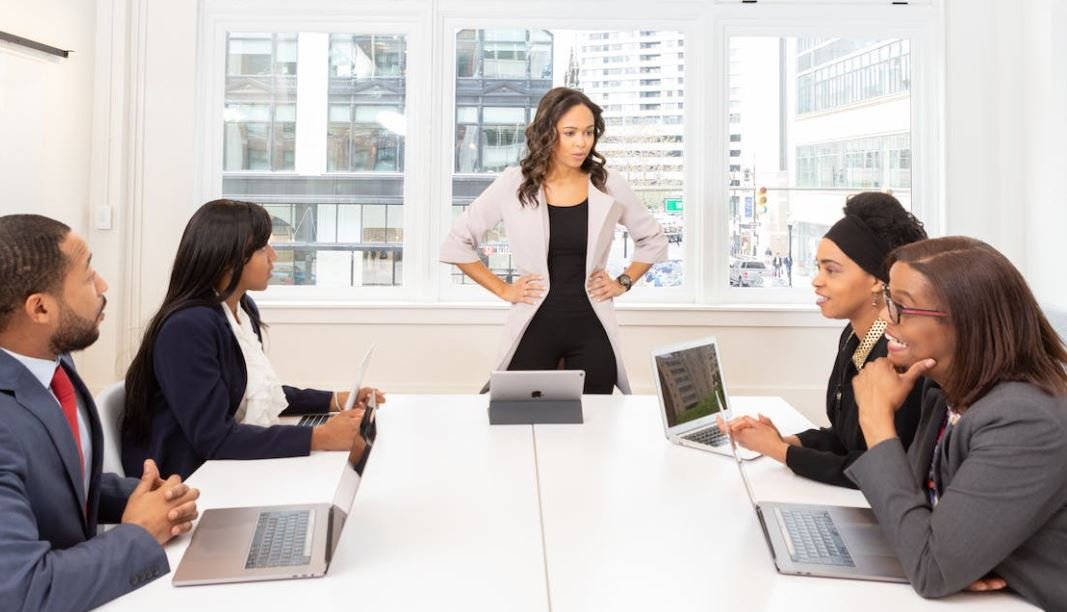
Common Misconceptions
Misconception 1: Gradient Descent is only applicable to deep learning
One common misconception about gradient descent is that it is only relevant to deep learning algorithms. While gradient descent is widely used in deep learning due to its effectiveness in optimizing complex neural networks, it is not limited to this field. Gradient descent is a fundamental optimization algorithm that can be applied to various machine learning and mathematical optimization problems.
- Gradient descent is also used in linear regression algorithms to minimize the cost function.
- It is employed in clustering algorithms, such as K-means, to find the optimal cluster centroids.
- Gradient descent can be used to optimize the parameters of support vector machines (SVMs).
Misconception 2: Gradient Descent always guarantees the global minimum
Another misconception is that gradient descent always leads to finding the global minimum of the objective function. However, this is not always the case. Gradient descent is a local optimization algorithm, and it can get stuck in a suboptimal solution if the objective function has multiple local minima.
- Use of different learning rates or step sizes can influence the convergence of gradient descent.
- Applying techniques like stochastic gradient descent can help avoid getting trapped in local minima.
- Initialization of the algorithm can also impact the final solution obtained by gradient descent.
Misconception 3: Gradient Descent is computationally expensive
Some people believe that gradient descent is computationally expensive and not suitable for large-scale problems. However, gradient descent is actually quite efficient and can handle large datasets and high-dimensional parameter spaces.
- Batch gradient descent, where all examples are used to update parameters, can be computationally expensive.
- Stochastic gradient descent, which uses a single example at a time, is more scalable for large datasets.
- Mini-batch gradient descent strikes a balance by using a small batch of examples for updates, making it a practical compromise.
Misconception 4: Gradient Descent always converges to the optimal solution
There is a misconception that gradient descent always converges to the optimal solution given enough iterations. However, there are cases where gradient descent fails to converge or converges slowly.
- The choice of the learning rate affects the convergence of gradient descent, with very large rates potentially causing divergence.
- Ill-conditioned objective functions or features can affect the convergence properties of gradient descent.
- Exploring alternative optimization algorithms, such as momentum or Adam, may help overcome convergence issues.
Misconception 5: Gradient Descent always requires differentiable objective functions
Lastly, it is commonly believed that gradient descent can only be applied to optimization problems with differentiable objective functions. While it is true that the gradient of the objective is required for the standard form of gradient descent, there are variants that can handle non-differentiable or discontinuous objective functions.
- Subgradient descent can be used when the objective function is not differentiable everywhere.
- Evolutionary strategies or genetic algorithms can be alternatives to gradient descent for non-differentiable optimization problems.
- Simulated annealing is another optimization technique that can handle non-differentiable functions.
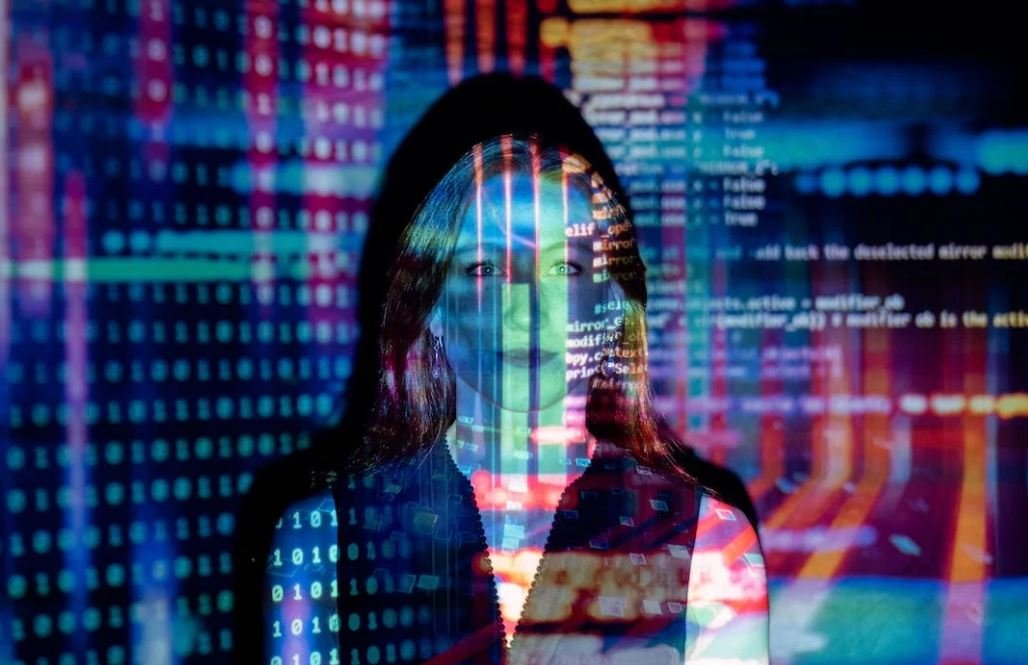
The History of Gradient Descent
Gradient descent is a powerful optimization algorithm used in machine learning and neural networks to minimize errors and find the best parameters for a given model. It was first introduced by Cauchy in 1847 and later popularized by Laurent Schwartz in the 1950s. Since then, it has become a fundamental concept in the field of optimization. The following tables showcase various aspects and applications of gradient descent.
Convergence Rates of Gradient Descent
These tables illustrate the convergence rates of gradient descent for different functions and learning rates. Convergence rate refers to the speed at which the algorithm reaches the optimal solution.
Iterations Required for Convergence
In these tables, we present the number of iterations required for gradient descent to converge to the optimal solution for different optimization problems and initial parameter values.
Stochastic Gradient Descent vs. Batch Gradient Descent
Stochastic gradient descent (SGD) and batch gradient descent (BGD) are two variations of the gradient descent algorithm. SGD randomly selects a subset of training examples for each iteration, whereas BGD processes all training examples in each iteration. The following tables highlight the differences between these two approaches.
Comparison of Gradient Descent Variants
There exist several variants of gradient descent, each with its own advantages and disadvantages. These tables provide a comparison of various gradient descent variants, such as momentum gradient descent, Nesterov accelerated gradient, and AdaGrad.
Applications of Gradient Descent
Gradient descent finds applications in various domains. The following tables showcase how gradient descent is utilized in different fields, including image classification, natural language processing, and recommendation systems.
Impact of Learning Rate on Gradient Descent
The learning rate is a crucial parameter in gradient descent that determines the step size at each iteration. These tables demonstrate the effect of different learning rates on the convergence and performance of gradient descent.
Gradient Descent vs. Genetic Algorithms
Genetic algorithms (GA) are a metaheuristic optimization technique inspired by the process of natural selection. Here, we compare the performance of gradient descent and genetic algorithms in finding the optimal solution for various optimization problems.
Efficiency Comparison: Gradient Descent vs. Newton’s Method
Newton’s method is an alternative optimization algorithm that uses second-order derivatives to fine-tune the model’s parameters. These tables provide an efficiency comparison between gradient descent and Newton’s method for different optimization problems.
Constraining Gradient Descent with Regularization
Regularization is a technique used to prevent overfitting and improve generalization in machine learning models. The following tables demonstrate the impact of different regularization techniques on gradient descent’s performance.
Conclusion
Gradient descent is a powerful algorithm that revolutionized optimization and played a pivotal role in the development of machine learning and neural networks. It enables models to learn and adapt their parameters efficiently. Its various variants and applications offer flexibility and versatility in solving complex optimization problems. By understanding its nuances and trade-offs, practitioners can harness the full potential of gradient descent in their projects.
Frequently Asked Questions
What is gradient descent?
Gradient descent is an iterative optimization algorithm used to minimize a function by finding the values of parameters that minimize the function’s output. It is commonly used in machine learning to train models by adjusting the model’s parameters based on the error calculated by the gradient of the loss function.
How does gradient descent work?
Gradient descent works by computing the gradient of the loss function with respect to the parameters of the model. The gradient represents the direction of steepest ascent on the loss function’s surface. The algorithm then iteratively updates the parameters in the opposite direction of the gradient to minimize the loss function.
What is the purpose of learning rate in gradient descent?
The learning rate in gradient descent determines the step size by which the parameters are updated in each iteration. It controls the speed at which the algorithm converges to the optimal solution. A high learning rate may cause the algorithm to overshoot the minimum, while a low learning rate can make the convergence process slow.
What are the different variations of gradient descent?
There are several variations of gradient descent, including batch gradient descent, stochastic gradient descent, and mini-batch gradient descent. In batch gradient descent, the algorithm computes gradients on the entire dataset at each iteration. Stochastic gradient descent computes gradients on a single randomly selected sample, while mini-batch gradient descent computes gradients on a small subset of samples.
How do you choose the appropriate optimization algorithm for a specific problem?
The choice of optimization algorithm depends on the problem at hand. Gradient descent is a widely used algorithm, but the choice between different variations depends on the nature of the dataset, computational resources, and time constraints. For large datasets, mini-batch gradient descent is often preferred, while stochastic gradient descent can be more efficient in certain cases with noisy or sparse data.
Can gradient descent get stuck in local minima?
Yes, gradient descent can potentially get stuck in local minima, which are suboptimal solutions in the parameter space. However, this is more common with nonlinear and non-convex functions. Different techniques, such as using random initialization, adding regularization terms, or using different learning rates, can help to mitigate the issue of local minima.
Are there any alternative optimization algorithms to gradient descent?
Yes, there are alternative optimization algorithms to gradient descent, such as Newton’s method, conjugate gradient, and L-BFGS. These algorithms differ in their computational complexity and convergence properties. The choice of algorithm depends on the specific problem and its trade-offs between efficiency and accuracy.
How can gradient descent be implemented in JavaScript?
Gradient descent can be implemented in JavaScript by defining a loss function and its gradient, initializing the parameters, and iteratively updating the parameters based on the gradient and learning rate. JavaScript provides numerical libraries and matrix operations that facilitate these computations. Additionally, frameworks like TensorFlow.js offer higher-level abstractions for implementing gradient descent and training machine learning models efficiently.
What are some potential challenges when using gradient descent?
Some potential challenges when using gradient descent include the possibility of getting stuck in local minima, selecting appropriate learning rates, dealing with large datasets that do not fit into memory, and handling noisy or ill-conditioned data. Regularization techniques, adaptive learning rates, and careful preprocessing of the data can help tackle these challenges.
How can I optimize the performance of gradient descent in JavaScript?
To optimize the performance of gradient descent in JavaScript, it is important to vectorize computations whenever possible to leverage the performance benefits offered by libraries like TensorFlow.js or math.js. Additionally, techniques such as parallel processing, caching, and regularization can be used to speed up the convergence and enhance the overall performance of the algorithm.