Gradient Descent Backpropagation
Gradient descent backpropagation is a popular optimization algorithm used in machine learning to train artificial neural networks. It is responsible for updating the weights of the network to minimize the error between the predicted and actual output. This iterative process makes the network learn and improve its performance over time.
Key Takeaways
- Gradient descent backpropagation is an optimization algorithm used in machine learning.
- It is used to train artificial neural networks by updating their weights.
- The algorithm minimizes the error between predicted and actual outputs.
- Gradient descent backpropagation is an iterative process.
In gradient descent backpropagation, the algorithm calculates the gradient of the loss function with respect to each weight in the network. It then updates these weights by taking steps proportional to the negative of the gradient. This process continues until the algorithm converges to a minimum point, indicating the best set of weights for the neural network.
Gradient descent backpropagation is like a hiker descending a mountain by taking small steps in the direction of the steepest slope.
Algorithm Steps
- Initialize the weights of the neural network randomly.
- Feed input data through the network and compute the output.
- Calculate the error between the predicted and actual output.
- Adjust the weights of the network using the chain rule to calculate the gradient.
- Update the weights by taking a step proportional to the negative gradient.
- Repeat steps 2-5 until convergence or a predetermined number of iterations.
The performance of gradient descent backpropagation depends on various factors, such as the learning rate, the choice of activation functions, and the architecture of the neural network. Choosing appropriate values for these factors is crucial to ensure the algorithm converges efficiently and avoids getting stuck in local minima.
Gradient descent backpropagation is a delicate balance between exploration and exploitation, as the algorithm aims to find the global minimum of the error function.
Data Points
Activation Function | Advantages | Disadvantages |
---|---|---|
Sigmoid | Smooth output, useful for binary classification. | Prone to vanishing gradient problem. |
ReLU | Avoids vanishing gradient problem, computationally efficient. | Output can be non-zero even for negative inputs. |
Learning Rate | Impact |
---|---|
High | Fast convergence but risks overshooting the minimum point. |
Low | Slower convergence but less likely to overshoot the minimum point. |
Although gradient descent backpropagation is a powerful algorithm, it is not without its limitations. It may suffer from the vanishing gradient problem, where the gradients become extremely small, slowing down the learning process. Additionally, it requires a significant amount of labeled training data to perform well, which can be resource-intensive and time-consuming to obtain.
The vanishing gradient problem can hinder deep neural networks from effectively learning complex patterns in the data.
Implementation in Python
Here is a simple example of implementing gradient descent backpropagation in Python:
import numpy as np
# Define the neural network architecture and activation function
# ...
# Initialize weights randomly
weights = np.random.randn(hidden_units, output_units)
# Training loop
for i in range(epochs):
# Feed input data through the network
# ...
# Compute the output and error
# ...
# Update the weights using gradient descent
# ...
# Evaluate the trained network
# ...
With this implementation, you can train a neural network using gradient descent backpropagation on your own dataset.
Gradient descent backpropagation is a fundamental algorithm in the field of machine learning. Its ability to update the weights of neural networks to minimize error makes it a powerful tool for training models. By understanding the key concepts and considerations behind this algorithm, you can effectively apply it to solve a wide range of machine learning problems.
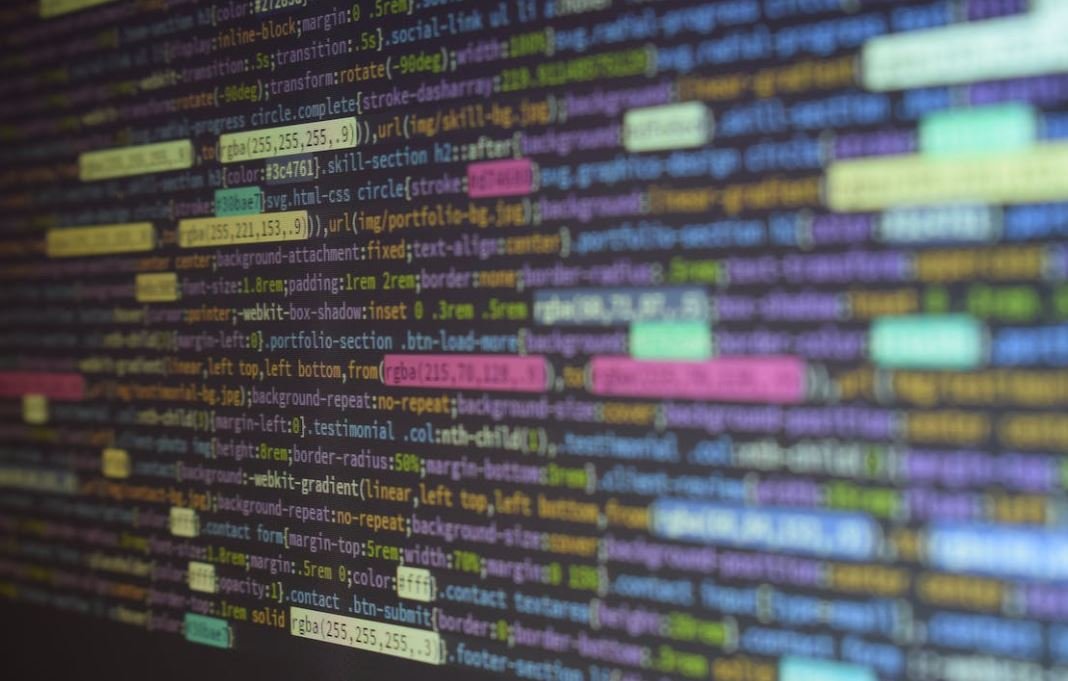
Common Misconceptions
Misconception 1: Gradient Descent is only used for training neural networks
One common misconception about gradient descent is that it is exclusively used for training neural networks. While it is true that gradient descent is a popular optimization algorithm in the context of deep learning, it is also widely applicable in other domains. For instance:
- Gradient descent can be used in linear regression to find the optimal parameters of a linear model.
- Gradient descent can be utilized in support vector machines to determine the hyperplane that best separates the data.
- Gradient descent is employed in collaborative filtering algorithms to optimize recommendations.
Misconception 2: Gradient Descent always converges to the global minimum
Another misconception is that gradient descent always converges to the global minimum of the optimization problem. However, this is not necessarily true, especially in non-convex optimization problems. Here are a few reasons why this misconception arises:
- Gradient descent may get stuck in a local minimum that is not the global minimum.
- The learning rate used in gradient descent could be too large, causing it to overshoot and miss the global minimum.
- If the initial starting point for gradient descent is far from the global minimum, it may not converge to the global minimum.
Misconception 3: Backpropagation is only used in deep learning
Backpropagation, the algorithm commonly used to compute gradients in neural networks, is often mistakenly thought to be exclusive to deep learning. However, backpropagation can be employed in other types of models as well. For example:
- Backpropagation can be utilized in feedforward neural networks with a single hidden layer.
- Backpropagation can be applied in recurrent neural networks (RNNs) for sequential data analysis.
- Backpropagation can be used in convolutional neural networks (CNNs) for image processing tasks.
Misconception 4: Gradient Descent always finds the optimal solution
It is important to note that gradient descent does not guarantee finding the optimal solution for every problem. Here are a few reasons why gradient descent may not converge to the optimal solution:
- If the optimization problem has multiple local minima, gradient descent may converge to a suboptimal solution.
- In the case of saddle points, where the gradient is close to zero but the point is not a minimum, gradient descent can get stuck.
- Gradient descent may struggle with high-dimensional optimization problems due to issues like the vanishing gradient problem.
Misconception 5: Backpropagation is computationally expensive
Backpropagation is sometimes falsely considered to be computationally expensive due to the commonly held belief that it involves calculating gradients for all the parameters in the neural network. However, backpropagation is an efficient algorithm, and modern implementations leverage matrix operations for parallelization to speed up computations. Additionally:
- Backpropagation can take advantage of GPU acceleration to further enhance its computational efficiency.
- The computational cost of backpropagation is primarily dependent on the size of the neural network rather than the specific type of operation being performed.
- Optimizations like mini-batch training can be used to reduce the computational burden of backpropagation.
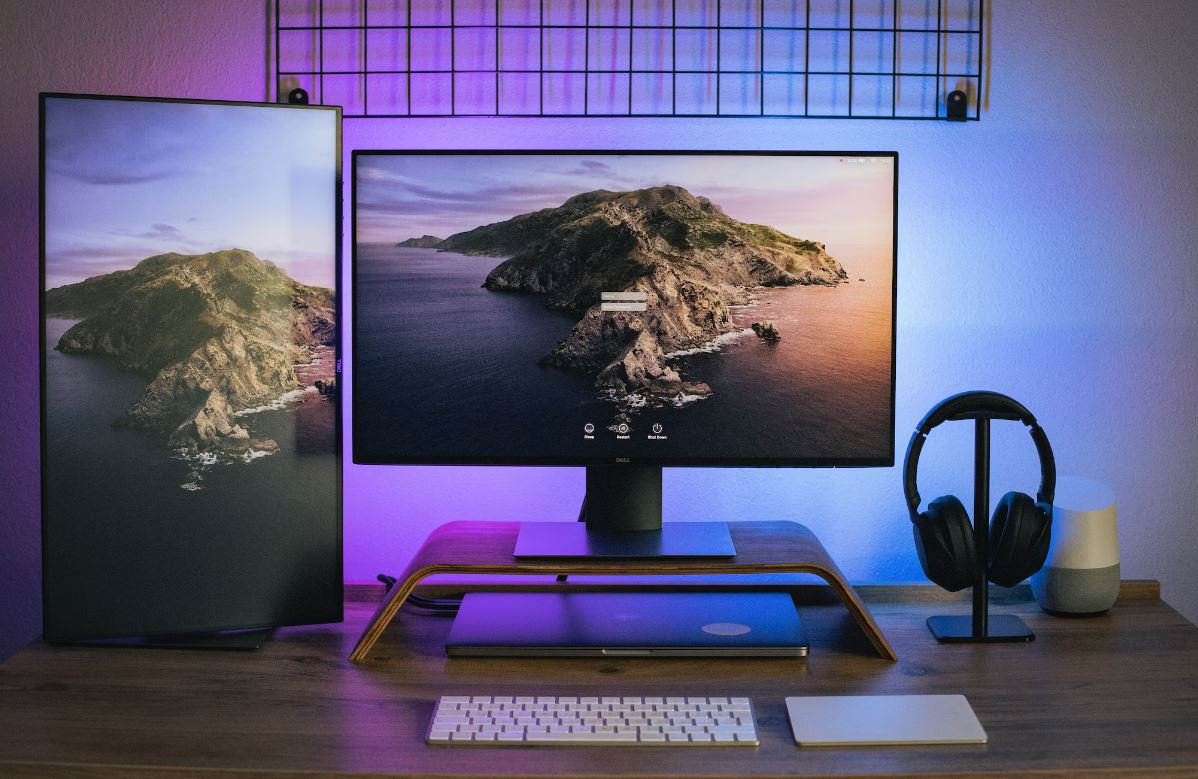
Introduction
This article explores the concept of Gradient Descent Backpropagation, an algorithm used in machine learning to train artificial neural networks. The method iteratively adjusts the network’s weights and biases to minimize the error of its output predictions. In the following tables, we present various aspects and components related to Gradient Descent Backpropagation, providing insightful information and relevant data for a comprehensive understanding of this technique.
Activation Functions
Activation functions play a vital role in neural networks by introducing non-linearity to enable complex mappings between the input and output. Here, we showcase different activation functions along with their respective formulas and key properties:
Activation Function | Formula | Range | Derivative |
---|---|---|---|
Sigmoid | 1 / (1 + e-x) | (0, 1) | f(x) * (1 – f(x)) |
ReLU | max(0, x) | [0, ∞) | 1 if x > 0, 0 if x ≤ 0 |
Tanh | (ex – e-x) / (ex + e-x) | (-1, 1) | 1 – f(x)2 |
Loss Functions
Loss functions quantify the discrepancy between predicted and actual output values, providing feedback to improve our model. The tables below present a selection of commonly used loss functions:
Loss Function | Formula | Key Characteristics |
---|---|---|
Mean Squared Error (MSE) | Σ(ypredicted – yactual)2 / n | Sensitive to outliers |
Binary Cross Entropy | -Σ(yactual * log(ypredicted) + (1 – yactual) * log(1 – ypredicted)) | Commonly used for binary classification |
Categorical Cross Entropy | -Σ(yactual * log(ypredicted)) | Used for multi-class classification |
Learning Rate Schedules
The learning rate determines the step size at each iteration during gradient descent, influencing the convergence speed and the quality of the final model. The following tables showcase different learning rate schedules, each outlining the progression of the learning rate over time:
Learning Rate Schedule | Description | Visual Representation |
---|---|---|
Constant | Learning rate remains fixed throughout training. | [——-] |
Time-Based Decay | Learning rate reduces linearly over time. | [//—–] |
Exponential Decay | Learning rate exponentially decays over time. | [\\\\\\] |
Regularization Techniques
To prevent overfitting and improve generalization, regularization techniques are applied. Here, we illustrate different regularization techniques along with their characteristics:
Regularization Technique | Description | Key Characteristics |
---|---|---|
L1 Regularization (Lasso) | Penalizes the absolute weights, encouraging sparsity. | Feature selection and interpretability |
L2 Regularization (Ridge) | Penalizes the squared weights, pushing them towards zero. | Reduces impact of irrelevant features |
Elastic Net | Combination of L1 and L2 regularization techniques. | Balance between feature selection and coefficient shrinkage |
Gradient Descent Optimization Algorithms
Gradient Descent Optimization Algorithms enhance the efficiency and effectiveness of the original gradient descent algorithm. The tables below showcase popular optimization algorithms and their key properties:
Optimization Algorithm | Description | Purpose |
---|---|---|
Stochastic Gradient Descent (SGD) | Updates weights and biases after each sample. | Efficient on large datasets |
Momentum | Accumulates past gradients to accelerate convergence. | Enhancing convergence speed |
Adam | Adaptive algorithm combining RMSprop and Momentum techniques. | Fast convergence and adaptivity |
Neural Network Architectures
The architecture of a neural network defines its structure and connectivity between layers. Here, we present different neural network architectures along with their properties:
Neural Network Architecture | Description | Use Cases |
---|---|---|
Feedforward Neural Network (FNN) | Information flows in a single, forward direction. | Image classification |
Recurrent Neural Network (RNN) | Allows information to persist throughout the network. | Speech recognition |
Convolutional Neural Network (CNN) | Specialized for image processing and analysis. | Object detection |
Backpropagation Algorithm
The backpropagation algorithm facilitates the calculation of gradients required for weight and bias updates. Understanding the steps involved in this algorithm is crucial for proper neural network training. Below, you’ll find the key steps of backpropagation:
Step | Description |
---|---|
Forward Pass | The input propagates through the network, producing predictions. |
Calculate Loss | Measure the discrepancy between predicted and actual values. |
Backward Pass | Gradients are calculated by applying the chain rule. |
Weight and Bias Update | Adjust the weights and biases based on the calculated gradients. |
Conclusion
Gradient Descent Backpropagation serves as a fundamental technique for training neural networks. From activation functions and loss functions to learning rate schedules and regularization techniques, each component plays a crucial role in optimizing model performance. Moreover, the exploration of optimization algorithms and neural network architectures enhances the effectiveness and efficiency of the training process. By utilizing backpropagation, we can leverage the power of gradient descent to fine-tune the weights and biases, resulting in neural networks capable of accurate predictions and learning complex patterns.
Frequently Asked Questions
What is Gradient Descent Backpropagation?
Gradient descent backpropagation is an iterative optimization algorithm used in artificial neural networks for updating the weights of the network’s connections to minimize the error between the predicted output and the actual output.
How does Gradient Descent Backpropagation work?
Gradient descent backpropagation works by first making forward passes through the neural network to obtain the predicted output. Then, it calculates the gradient of the loss function with respect to the network’s weights. Finally, it updates the weights in the opposite direction of the gradient to minimize the loss.
What is the purpose of Gradient Descent Backpropagation?
The purpose of gradient descent backpropagation is to train the neural network by iteratively adjusting its weights to improve the accuracy of the predicted output. It allows the network to learn from labeled training data and generalize to make predictions on unseen data.
What is the difference between Gradient Descent and Backpropagation?
Gradient descent refers to the optimization algorithm used to update the network’s weights, while backpropagation is the process of calculating the gradients of the loss function with respect to the weights. Gradient descent backpropagation combines both of these techniques.
What is the loss function used in Gradient Descent Backpropagation?
The loss function used in gradient descent backpropagation can vary depending on the task. For regression problems, the mean squared error (MSE) loss function is commonly used, while for classification problems, the cross-entropy loss function is often employed.
What are the advantages of Gradient Descent Backpropagation?
Gradient descent backpropagation allows the neural network to efficiently learn complex patterns and relationships in the data. It is capable of handling large datasets, generalizing to unseen instances, and can be applied to various types of problems, including both regression and classification tasks.
What are the limitations of Gradient Descent Backpropagation?
Gradient descent backpropagation may suffer from the issues of local minima and getting stuck in plateaus, where the algorithm cannot further improve the model’s performance. It also requires determining appropriate hyperparameters, such as the learning rate, and may be computationally expensive for deep neural networks.
Are there alternatives to Gradient Descent Backpropagation?
Yes, there are alternative optimization algorithms that can be used to update the weights of a neural network, such as stochastic gradient descent (SGD), Adam, and Adagrad. Additionally, there are various variations of backpropagation, including resilient backpropagation (RPROP) and conjugate gradient descent.
Are there any prerequisites for implementing Gradient Descent Backpropagation?
Prior knowledge in machine learning and neural networks is helpful for implementing gradient descent backpropagation. Understanding concepts like activation functions, loss functions, and the basics of neural network architecture will greatly assist in successfully implementing the algorithm.
How can one evaluate the performance of a model trained using Gradient Descent Backpropagation?
The performance of a model trained with gradient descent backpropagation can be evaluated using various evaluation metrics based on the problem at hand. For classification tasks, metrics such as accuracy, precision, recall, and F1-score can be used. For regression tasks, metrics like mean absolute error (MAE) and root mean squared error (RMSE) are commonly employed.