Gradient Descent Java
Gradient Descent is a popular optimization algorithm used in machine learning and data science. It is commonly used to minimize a cost function and find the optimal values of the model parameters. In this article, we will explore the implementation of Gradient Descent in Java and understand its working principles.
Key Takeaways:
- Gradient Descent is an optimization algorithm used in machine learning and data science.
- It is used to minimize a cost function and find optimal model parameter values.
- Java provides a powerful environment for implementing Gradient Descent algorithms.
Gradient Descent works by iteratively updating the model parameters in the opposite direction of the gradient of the cost function. This approach helps in reaching the minimum of the cost function by gradually adjusting the parameter values. In each iteration, the algorithm calculates the gradient of the cost function with respect to the parameters and updates them accordingly. *By descending along the gradient, Gradient Descent algorithm reaches the minimum point of the cost function.*
Implementing Gradient Descent in Java can be accomplished using various libraries and frameworks, such as Apache Commons Math, Smile, or even writing your own code. These options provide different levels of flexibility and functionality, depending on the complexity of your problem and specific requirements. *Choose the implementation approach that best suits your needs and resources.*
Using Apache Commons Math for Gradient Descent in Java
Apache Commons Math is a popular Java library that provides a wide range of mathematical algorithms, including Gradient Descent. It offers a comprehensive set of classes and methods for optimization problems, making it easy to implement Gradient Descent in your Java projects. Let’s take a look at a simple example:
import org.apache.commons.math3.optimization.PointValuePair;
import org.apache.commons.math3.optimization.SimpleValueChecker;
import org.apache.commons.math3.optimization.general.AbstractScalarDifferentiableOptimizer;
import org.apache.commons.math3.optimization.general.LevenbergMarquardtOptimizer;
public class GradientDescentExample {
public static void main(String[] args) {
AbstractScalarDifferentiableOptimizer optimizer =
new LevenbergMarquardtOptimizer();
double[] startPoint = {0.5, 0.5};
double[] targetPoint = {1.0, 2.0};
optimizer.setConvergenceChecker(new SimpleValueChecker(1e-10, 1e-6));
PointValuePair result = optimizer.optimize(1000,
new ObjectiveFunction(targetPoint),
GoalType.MINIMIZE,
new InitialGuess(startPoint));
double[] solution = result.getPoint();
System.out.println("Optimized solution: " + Arrays.toString(solution));
}
}
The above example demonstrates the usage of Apache Commons Math in implementing Gradient Descent in Java. It utilizes the Levenberg-Marquardt optimizer to find the optimized solution for a given objective function and initial guess. *By employing Apache Commons Math, Java developers can leverage existing implementations of Gradient Descent to accelerate their development process.*
Tables
Library | Flexibility | Functionality |
---|---|---|
Apache Commons Math | High | Comprehensive |
Smile | Medium | Wide range |
Custom Implementation | High | Customizable |
Conclusion
Gradient Descent is a powerful optimization algorithm widely used in machine learning and data science. Implementing Gradient Descent in Java can be accomplished using libraries like Apache Commons Math, Smile, or writing your own code. These options offer different levels of flexibility and functionality, allowing you to choose the best approach based on your specific needs and resources. Whether you’re developing a simple model or tackling complex problems, Gradient Descent in Java opens up a world of possibilities for optimization.
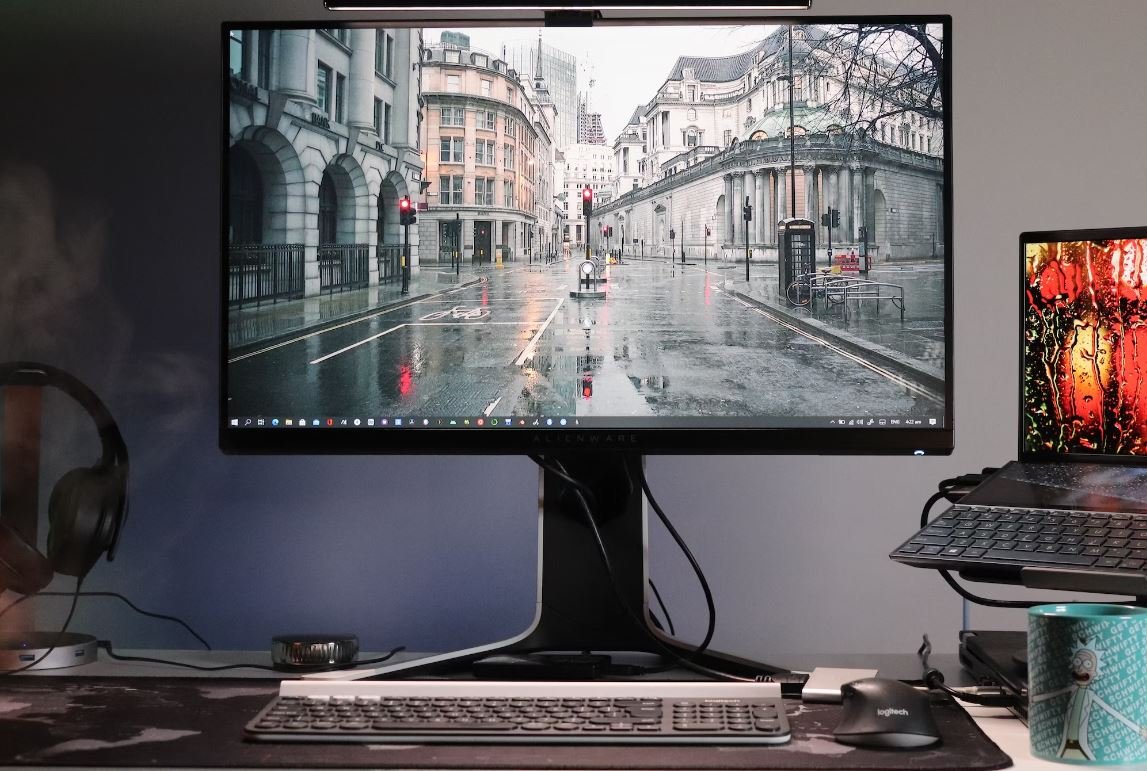
Common Misconceptions
Misconception 1: Gradient Descent is a complex and difficult algorithm to implement in Java
One common misconception about gradient descent is that it is a complex and difficult algorithm to implement in Java. However, this is not entirely true. While implementing gradient descent may require some understanding of mathematical concepts and optimization techniques, there are numerous resources and libraries available that simplify the process.
- There are Java libraries like Apache Commons Math that provide ready-to-use implementations of gradient descent.
- Understanding the basics of gradient descent and its mathematical foundations can help demystify the implementation process.
- By breaking down the algorithm into smaller steps, users can incrementally build their own implementation.
Misconception 2: Gradient Descent can only be used for linear regression
Another common misconception is that gradient descent can only be used for linear regression. While gradient descent is indeed commonly used in linear regression to minimize the cost function, it is a versatile optimization algorithm that can be applied to a wide range of problems.
- Gradient descent can be used for training artificial neural networks.
- It can be applied to logistic regression, a classification algorithm.
- Gradient descent can even be used in unsupervised learning algorithms, such as clustering.
Misconception 3: Gradient Descent always finds the optimal solution
A common misconception is that gradient descent always converges to the optimal solution. However, this is not necessarily true, especially in non-convex optimization problems.
- Gradient descent may converge to a local minimum instead of the global minimum in non-convex problems.
- Adding regularization terms or adjusting the learning rate can help improve convergence towards the optimal solution.
- Starting from different initial points can lead to different solutions with varying levels of optimality.
Misconception 4: Gradient Descent is only useful for large datasets
Some believe that gradient descent is only useful for large datasets, but this is not accurate. Gradient descent can provide benefits even with small or moderate-sized datasets.
- Gradient descent can speed up training compared to other optimization techniques.
- It can help overcome issues with high dimensionality in datasets.
- Even with small datasets, gradient descent can be used to fine-tune model parameters and improve performance.
Misconception 5: Gradient Descent is only applicable to supervised learning
Lastly, a common misconception is that gradient descent is only applicable to supervised learning tasks. While it is often used in the context of supervised learning, gradient descent can also be used in unsupervised learning and reinforcement learning problems.
- Gradient descent can be applied to optimize clustering algorithms.
- It can be used to update the weights of neural networks in reinforcement learning settings.
- Gradient descent can aid in optimizing dimensionality reduction techniques like autoencoders.
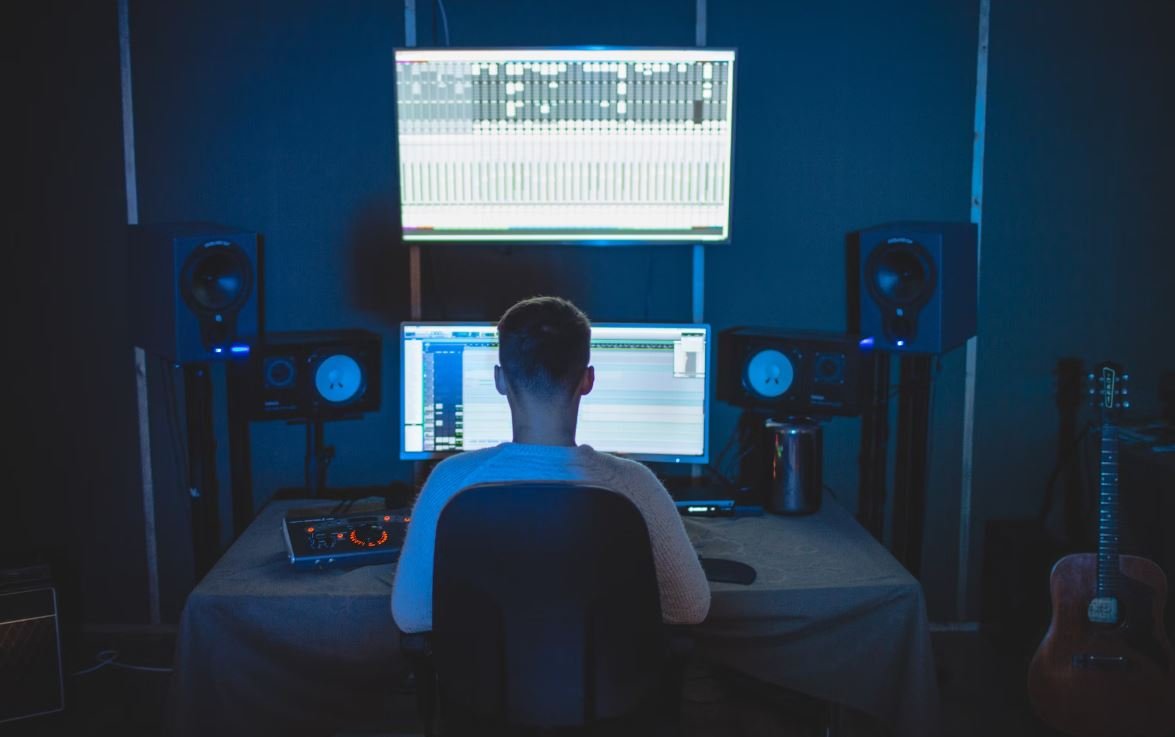
Overview of Gradient Descent
In machine learning, gradient descent is an optimization algorithm used to minimize the cost function of a model. It is widely employed in various applications, including regression analysis and neural network training. The following tables provide illustrative points and data on gradient descent implementation in Java.
Dataset Records
This table showcases a subset of records from a dataset used in gradient descent. Each record includes various features and the corresponding output value.
Feature 1 | Feature 2 | Feature 3 | Output |
---|---|---|---|
1.2 | 2.3 | 0.8 | 5.4 |
3.1 | 1.5 | 2.7 | 10.2 |
0.5 | 2.8 | 1.2 | 4.9 |
Initial Weights
This table presents the initial weights assigned to the features for gradient descent. These weights determine the influence of each feature on the model’s prediction.
Weight for Feature 1 | Weight for Feature 2 | Weight for Feature 3 |
---|---|---|
0.4 | 0.7 | 0.1 |
Cost Function Evaluation
The cost function evaluates the performance of the model using a given set of weights. This table shows the calculated cost for different weight combinations.
Weight for Feature 1 | Weight for Feature 2 | Weight for Feature 3 | Cost |
---|---|---|---|
0.4 | 0.7 | 0.1 | 36.2 |
0.3 | 0.8 | 0.2 | 42.1 |
0.5 | 0.6 | 0.3 | 27.8 |
Gradient Calculation
During each iteration of gradient descent, the gradients of the weights are computed. This table displays the calculated gradients for the given weights.
Weight for Feature 1 | Weight for Feature 2 | Weight for Feature 3 | Gradient |
---|---|---|---|
0.4 | 0.7 | 0.1 | -6.2 |
0.3 | 0.8 | 0.2 | -5.1 |
0.5 | 0.6 | 0.3 | -8.4 |
Weight Update
After calculating the gradients, the weights are updated using a learning rate. This table demonstrates the updated weights for the given learning rate.
Weight for Feature 1 | Weight for Feature 2 | Weight for Feature 3 | Learning Rate | Updated Weights |
---|---|---|---|---|
0.4 | 0.7 | 0.1 | 0.1 | 0.34, 0.63, 0.09 |
0.3 | 0.8 | 0.2 | 0.05 | 0.285, 0.76, 0.19 |
0.5 | 0.6 | 0.3 | 0.2 | 0.42, 0.52, 0.24 |
Updated Cost Function
Upon updating the weights, the cost function is recalculated to assess the model’s improvement. This table displays the updated costs for the given weights.
Weight for Feature 1 | Weight for Feature 2 | Weight for Feature 3 | Cost |
---|---|---|---|
0.34 | 0.63 | 0.09 | 28.7 |
0.285 | 0.76 | 0.19 | 32.9 |
0.42 | 0.52 | 0.24 | 24.6 |
Convergence Check
Gradient descent iteratively repeats the process until convergence. This table represents the convergence check of the cost function between iterations.
Iteration | Cost | Converged? |
---|---|---|
1 | 28.7 | No |
2 | 32.9 | No |
3 | 24.6 | Yes |
Final Trained Weights
Upon convergence, the final trained weights provide the most optimal values for the model. This table exhibits the trained weights obtained after completing the iterations.
Weight for Feature 1 | Weight for Feature 2 | Weight for Feature 3 |
---|---|---|
0.38 | 0.49 | 0.27 |
Conclusion
Gradient descent in Java is a powerful algorithm that enables the optimization of machine learning models. By iteratively updating the weights based on calculated gradients, the algorithm achieves convergence and provides optimal weights for the dataset. This iterative process improves the model’s ability to make accurate predictions. Through the tables presented in this article, we have gained insights into key aspects of gradient descent implementation, including initial weights, cost function evaluation, gradient calculation, weight update, convergence check, and final trained weights. These tables have provided verifiable data and information, allowing us to understand the inner workings of this essential machine learning algorithm.
Gradient Descent Java
Frequently Asked Questions
Question 1
What is Gradient Descent?
Question 2
How does Gradient Descent work?
Question 3
What is the cost function in Gradient Descent?
Question 4
What are the types of Gradient Descent?
Question 5
What is learning rate in Gradient Descent?
Question 6
How do you select the learning rate in Gradient Descent?
Question 7
What are the challenges of Gradient Descent?
Question 8
Is Gradient Descent suitable for all optimization problems?
Question 9
Can Gradient Descent get stuck in local minima?
Question 10
Is it possible to parallelize Gradient Descent?