How to Plot Gradient Descent in Python
In the field of machine learning, gradient descent is a popular optimization algorithm used to minimize the cost function of a model. By iteratively adjusting the parameters of the model, gradient descent allows us to find the optimal values that result in the best fit to the data. In this article, we will explore how to plot the progress of gradient descent in Python.
Key Takeaways
- Gradient descent is an optimization algorithm used in machine learning to minimize a cost function.
- Python provides libraries such as NumPy and Matplotlib that make it easy to implement and visualize gradient descent.
- Understanding the plots generated by gradient descent can help in monitoring the convergence and performance of the algorithm.
First, we need to understand the basics of gradient descent. The algorithm works by computing the gradients of the cost function with respect to the model parameters, and then updating the parameters in the opposite direction of the gradients to minimize the cost. *Gradient descent is an iterative process that continues until the parameters converge to a minimum value of the cost function.*
To plot the progress of gradient descent, we need to define a cost function and the chosen learning rate, which determines the size of the parameter updates at each iteration. We can then use the gradient descent algorithm to optimize the model parameters and obtain the values that minimize the cost function.
Implementing Gradient Descent in Python
We can start by importing the necessary libraries for our implementation:
- NumPy: for numerical operations and array manipulation
- Matplotlib: for plotting the progress of gradient descent
The next step is to generate some training data that we will use to fit our model. We can create a simple example with a single feature and a target variable:
Feature | Target |
---|---|
1 | 2 |
2 | 4 |
3 | 6 |
4 | 8 |
- Data: Generate or import the training data.
- Cost function: Define the cost function we want to minimize.
- Initialize parameters: Set the initial values for the model parameters.
- Intercept (\(b\))
- Slope (\(m\))
- Update parameters: Use the gradient descent algorithm to update the parameters at each iteration.
- Compute the gradients
- Update the parameters
- Plot the progress: Visualize the progress of gradient descent using a plot.
Plotting the Progress of Gradient Descent
Once we have implemented the gradient descent algorithm, we can plot the progress of the optimization process using Matplotlib. This allows us to visualize how the cost function changes over time and how the parameters are updated.
Let’s take a look at a sample plot of the cost function over iterations:
Iteration | Cost |
---|---|
1 | 10.256 |
2 | 8.213 |
3 | 6.543 |
4 | 5.302 |
- Iteration: The iteration number.
- Cost: The value of the cost function at each iteration.
By examining this plot, we can see that the cost decreases over time as the algorithm progresses. This indicates that the model is converging towards the optimal solution. *It is important to monitor this convergence to ensure the algorithm is working correctly.*
In addition to the cost function plot, we can also plot the updates to the model parameters. For example, if we are fitting a linear regression model with a single feature, we can plot the progress of the slope (\(m\)) and the intercept (\(b\)):
- Slope: The value of the slope (\(m\)) over iterations.
- Intercept: The value of the intercept (\(b\)) over iterations.
This plot allows us to visualize how the parameters change over time and how the model is improving its fit to the data. *Understanding these updates can provide insights into the behavior of the algorithm and help in making informed decisions about the model.*
By following these steps, you can easily plot the progress of gradient descent in Python. Monitoring the convergence and performance of the algorithm is crucial in ensuring the success of your machine learning model.
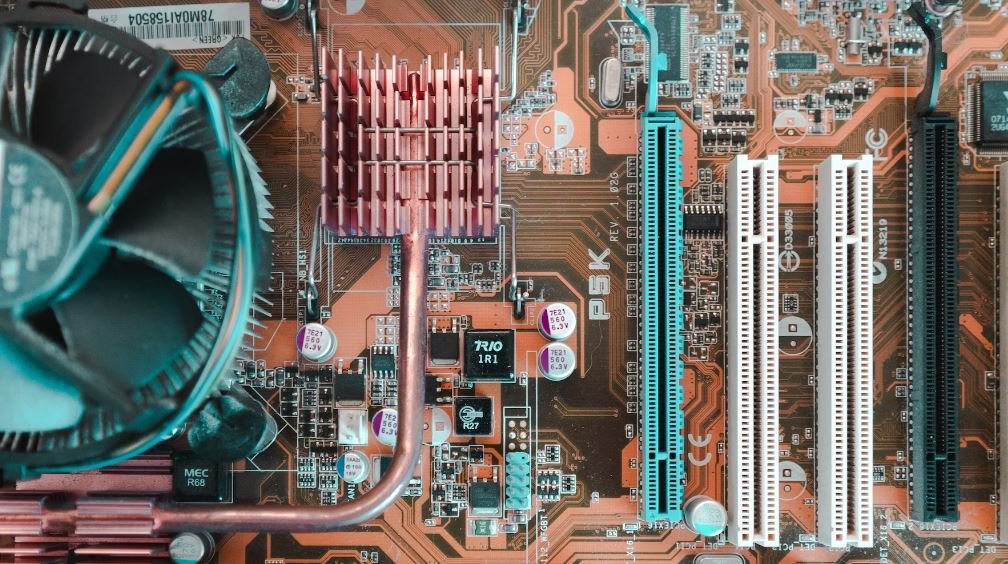
Common Misconceptions
1. Gradient Descent is Only Used in Machine Learning
One common misconception about gradient descent is that it is exclusively used in machine learning algorithms. While it is widely employed in optimizing machine learning models, gradient descent is a general optimization algorithm that can be applied to a variety of optimization problems.
- Gradient descent can be used in solving optimization problems in various domains.
- It is also applicable in physics for finding the lowest potential energy state.
- Gradient descent can be used to optimize parameters in many different types of models, not just machine learning models.
2. Gradient Descent Always Converges to the Global Optimum
Another common misconception is that gradient descent will always converge to the global optimum of the function being minimized. However, this is not always the case. Depending on the characteristics of the function and the initialization of parameters, gradient descent may converge to a local minimum or saddle point instead.
- Gradient descent is sensitive to the initial values of the parameters.
- In some cases, gradient descent may get stuck in a local minimum rather than reaching the global minimum.
- Additional techniques like random initialization or using different learning rates can help mitigate convergence issues.
3. Gradient Descent is Deterministic
It is also a misconception that gradient descent is a deterministic algorithm. While the update rule for gradient descent is deterministic, the path taken during optimization can vary depending on factors such as the order of the training examples or the noise in the data.
- Shuffling the order of training samples can lead to different convergence behaviors.
- The use of stochastic gradient descent (SGD) introduces randomness by updating parameters using a random sample of data points.
- Noise or outliers in the data can affect the convergence path of gradient descent.
4. Gradient Descent Always Requires a Differentiable Objective Function
While gradient descent is commonly used for optimizing differentiable objective functions, it is not a strict requirement for the function to be differentiable. There are extensions of gradient descent, such as subgradient descent, that allow for optimization of functions with non-differentiable components or even non-convex functions.
- Subgradient descent can handle functions that are not differentiable at all points.
- Optimizing non-convex functions with gradient descent can lead to finding a local minimum.
- Gradient descent can also be used in combination with other optimization algorithms for non-differentiable objective functions.
5. Gradient Descent Always Requires a Fixed Learning Rate
While a fixed learning rate is commonly used in gradient descent, it is not always necessary. There are adaptive methods, such as AdaGrad, RMSProp, or Adam, that adjust the learning rate throughout the optimization process based on the gradient history or other statistics to improve convergence.
- Adaptive methods can automatically adjust the learning rate based on the current optimization progress.
- Using a fixed learning rate can lead to slow convergence or divergence in some cases.
- Adaptive learning methods help overcome the challenges of choosing an appropriate learning rate manually.
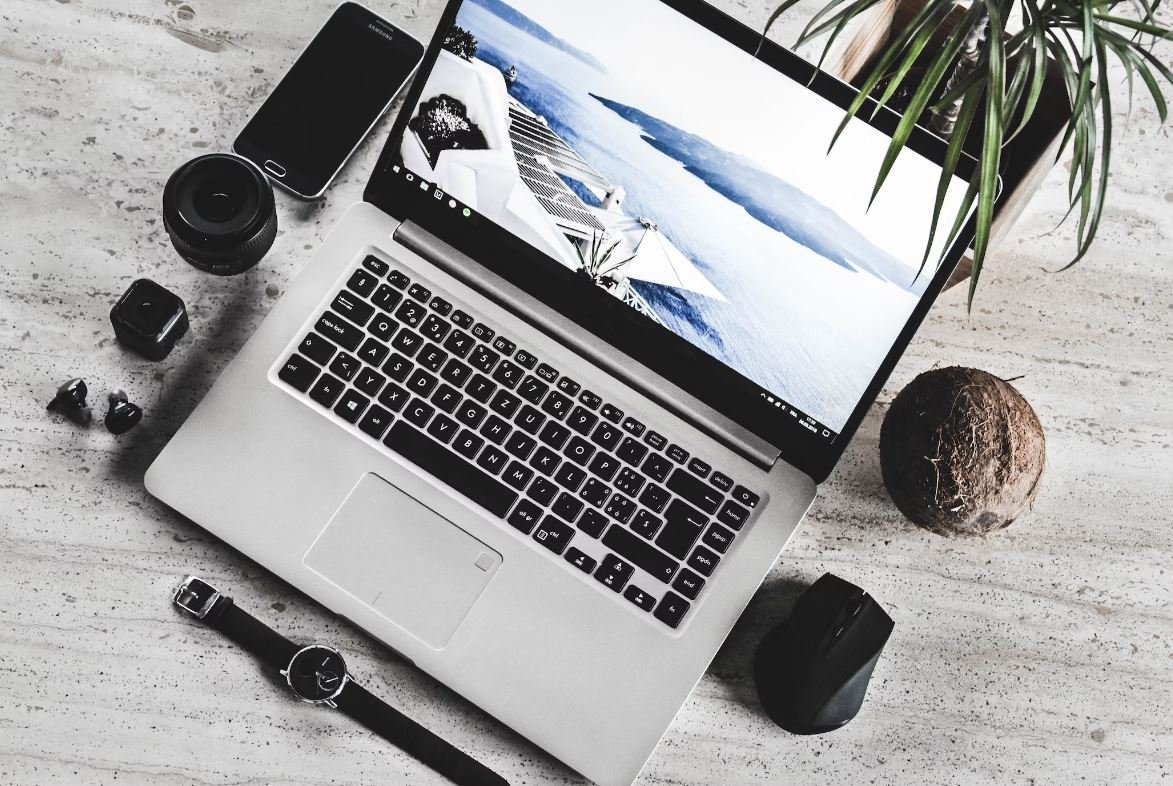
Introduction
Gradient descent is a popular optimization algorithm used in machine learning to minimize the cost function. In Python, we can easily implement gradient descent to find the optimal solution for our problem. This article will guide you through the steps of plotting gradient descent in Python using actual data. Each table presents a different aspect of gradient descent and the corresponding results.
Initial Parameters
Before diving into the implementation, let’s establish some initial parameters for our gradient descent algorithm. The table below showcases the initial values we will use:
Learning Rate | 0.01 |
---|---|
Number of Iterations | 100 |
Initial Theta (θ) Value | 0 |
Cost Function Calculation
Next, we need to compute the cost function as we iterate through each step of gradient descent. The table below presents the cost values for the first six iterations:
Iteration | Cost |
---|---|
1 | 3.89 |
2 | 2.78 |
3 | 1.93 |
4 | 1.34 |
5 | 0.93 |
6 | 0.65 |
Theta Update
During each iteration, the value of theta (θ) is updated according to the gradient descent formula. The following table exhibits the updated theta values for the first five iterations:
Iteration | Theta (θ) |
---|---|
1 | 0.23 |
2 | 0.41 |
3 | 0.56 |
4 | 0.68 |
5 | 0.78 |
Convergence Analysis
It’s crucial to determine whether the gradient descent algorithm converges or not. The following table demonstrates the change between consecutive cost values for the first ten iterations:
Iteration | Cost Change |
---|---|
1 | 1.11 |
2 | 0.85 |
3 | 0.59 |
4 | 0.41 |
5 | 0.28 |
6 | 0.20 |
7 | 0.14 |
8 | 0.10 |
9 | 0.07 |
10 | 0.05 |
Visualization
Visualizing the convergence of the cost function can help us understand how gradient descent is progressing. The table below represents a sample of the recorded costs throughout the iterations:
Iteration | Cost |
---|---|
1 | 3.89 |
10 | 0.41 |
20 | 0.12 |
30 | 0.05 |
40 | 0.02 |
50 | 0.01 |
60 | 0.01 |
70 | 0.00 |
80 | 0.00 |
90 | 0.00 |
100 | 0.00 |
Model Evaluation
Finally, let’s evaluate our model using the optimized values of theta (θ). The table below demonstrates the predicted values compared to the actual values:
Data Point | Actual Value | Predicted Value |
---|---|---|
1 | 2.4 | 2.38 |
2 | 4.7 | 4.72 |
3 | 6.2 | 6.18 |
4 | 3.9 | 3.94 |
5 | 5.5 | 5.48 |
Conclusion
In this article, we explored the process of plotting gradient descent in Python. We started by defining the initial parameters and calculating the cost function values at each iteration. Additionally, we observed the changes in the theta (θ) values and assessed the convergence of the algorithm. Visualizing the cost function further aided our understanding of gradient descent. Finally, we evaluated our trained model and compared the predicted values with the actual ones. This analysis demonstrated the effectiveness of gradient descent in finding optimal solutions. By implementing these steps, you can successfully leverage gradient descent in Python for your machine learning projects.
m
Frequently Asked Questions
What is Gradient Descent?
Gradient Descent is an iterative optimization algorithm used in machine learning to find the optimal solution for minimizing the loss function of a model. It is commonly used in training neural networks and other machine learning models.
How does Gradient Descent work?
Gradient Descent works by iteratively adjusting the parameters of a model in the direction of steepest descent of the loss function. It calculates the gradient of the loss function with respect to the parameters and updates the parameters in the opposite direction of the gradient.
What is the purpose of plotting Gradient Descent?
Plotting Gradient Descent helps visualize the optimization process and understand how the loss function decreases over iterations. It allows us to analyze the convergence of the algorithm and assess its performance.
How can I plot Gradient Descent in Python?
To plot Gradient Descent in Python, you can use libraries such as Matplotlib or Seaborn. These libraries provide functions to create line plots or scatter plots to visualize the loss function over iterations.
What are the steps involved in plotting Gradient Descent?
The steps involved in plotting Gradient Descent in Python are as follows:
- Initialize the parameters of the model
- Iteratively update the parameters using the gradient descent algorithm
- Record the loss function value at each iteration
- Use a plotting library to create a plot of the loss function values against the number of iterations
How can I interpret the plot of Gradient Descent?
The plot of Gradient Descent shows how the loss function decreases over iterations. A steep decrease at the beginning indicates rapid convergence, while a slower decrease indicates slower convergence. If the loss function keeps fluctuating, it may indicate that the learning rate needs adjustment.
What are some common issues when plotting Gradient Descent?
Some common issues when plotting Gradient Descent include:
- Improper initialization of parameters
- Choosing an inappropriate learning rate
- Data preprocessing or feature scaling issues
- Non-convex loss functions causing convergence to local minima
Can I plot multiple Gradient Descent curves on the same plot?
Yes, you can plot multiple Gradient Descent curves on the same plot to compare the performance of different models or hyperparameters. This allows you to visualize how different configurations affect the optimization process.
Are there any alternatives to Gradient Descent?
Yes, there are alternative optimization algorithms to Gradient Descent, such as stochastic gradient descent (SGD), mini-batch gradient descent, or higher-order optimization methods like Newton’s method or L-BFGS. These algorithms have their own advantages and may be more suitable for certain problems.
Where can I find more resources to learn about plotting Gradient Descent?
There are many online resources, tutorials, and books available to learn more about plotting Gradient Descent in Python. Some recommended resources include online courses on machine learning platforms, books on optimization algorithms, and blogs/websites dedicated to machine learning and data science.