Gradient Descent in PyTorch
Gradient Descent is a widely used optimization algorithm in Machine Learning for minimizing the loss function of a model. In PyTorch, one of the most popular deep learning frameworks, gradient descent can be implemented efficiently using its built-in functionalities.
Key Takeaways
- Gradient Descent is used to minimize the loss function in Machine Learning models.
- PyTorch provides efficient methods for implementing gradient descent.
- Learning rate and number of iterations are crucial parameters in gradient descent.
What is Gradient Descent?
Gradient Descent is an optimization algorithm used to minimize the loss function in Machine Learning models. It updates the parameters of the model iteratively in the direction of steepest descent of the loss function, gradually reaching the optimal solution.
Gradient Descent is like descending a mountain by taking the steepest path downhill.
Implementing Gradient Descent in PyTorch
In PyTorch, gradient descent can be implemented using the torch.optim package. This package provides various optimization algorithms, including the popular stochastic gradient descent (SGD) algorithm.
PyTorch makes implementing gradient descent easy and efficient for deep learning tasks.
Using the SGD Optimizer
The torch.optim.SGD class in PyTorch implements the SGD optimization algorithm. It requires the model parameters and the learning rate as input. Optionally, other parameters such as momentum and weight decay can be specified to enhance the optimization process.
- Learning rate (lr): Controls the step size at each iteration.
- Momentum (momentum): Helps accelerate the convergence by accumulating gradients from previous iterations.
- Weight decay (weight_decay): Regularizes the model to prevent overfitting by applying a penalty on the parameters.
Example Implementation
Here’s an example of implementing gradient descent using the SGD optimizer in PyTorch:
import torch
import torch.optim as optim
# Create a model
model = MyModel()
# Define the loss function
criterion = ...
# Define the optimizer
optimizer = optim.SGD(model.parameters(), lr=0.01)
Training the Model
After setting up the optimizer, we can start training the model using gradient descent. This involves several iterations where we:
- Compute the predictions of the model given the input data.
- Calculate the loss by comparing the predictions with the actual labels.
- Clear the gradients of the optimizer to avoid accumulation from previous iterations.
- Compute the gradients of the loss with respect to the model parameters.
- Update the model parameters using the optimizer.
Tables
Learning Rate | Iterations |
---|---|
0.001 | 100 |
0.01 | 1000 |
Other Optimization Algorithms
PyTorch provides various optimization algorithms in addition to SGD. These include:
- Adam: Adaptive Moment Estimation
- AdaGrad: Adaptive Subgradient Descent
- RMSprop: Root Mean Square Propagation
Using the right optimizer for your model can significantly improve training performance.
Conclusion
Gradient Descent is a fundamental optimization algorithm used in Machine Learning. In PyTorch, implementing gradient descent is straightforward thanks to its built-in functionalities, such as the torch.optim package. By choosing the appropriate optimizer and tuning the learning rate and number of iterations, you can efficiently train your models and achieve better performance.
References
- PyTorch Documentation, https://pytorch.org/docs/stable/index.html
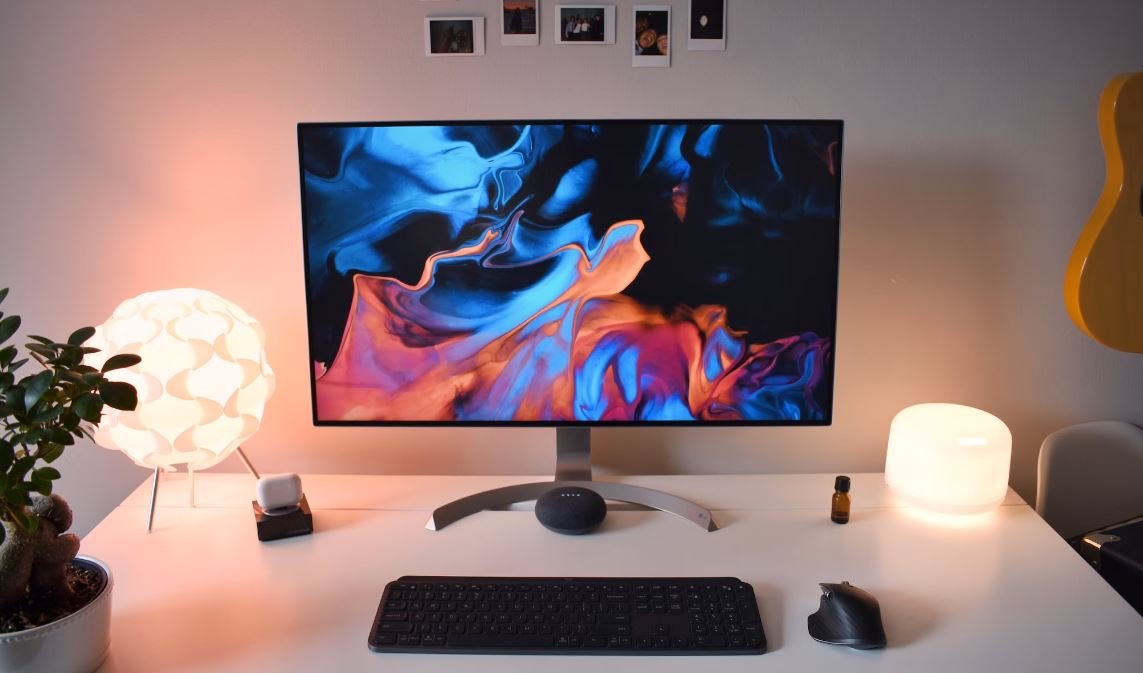
Common Misconceptions
1. Gradient Descent in PyTorch is only used for deep learning
- Gradient descent can be applied to a wide range of optimization problems, not just deep learning tasks.
- It is commonly used in areas such as machine learning, computer vision, natural language processing, and data science.
- PyTorch provides a powerful framework for implementing gradient descent algorithms in various domains.
One common misconception about Gradient Descent in PyTorch is that it is solely used for deep learning. While it is true that PyTorch is widely used in deep learning applications, gradient descent is a general optimization algorithm that can be applied to various types of problems. It is commonly used in fields such as machine learning, computer vision, natural language processing, and data science. PyTorch provides a robust framework for implementing gradient descent algorithms across these domains.
2. Gradient Descent in PyTorch always converges to the global minimum
- Gradient descent algorithms generally converge to a local or global minimum, but not necessarily the global minimum.
- The effectiveness of gradient descent depends on factors such as the chosen learning rate and the objective function landscape.
- In some cases, gradient descent may get trapped in local minima or saddle points, leading to suboptimal solutions.
Another misconception is that Gradient Descent in PyTorch always converges to the global minimum. While gradient descent is a powerful optimization algorithm, it does not guarantee reaching the global minimum in all scenarios. The algorithm primarily converges to a local minimum, which may not be the global minimum. The effectiveness of gradient descent is influenced by various factors such as the learning rate chosen and the landscape of the objective function. In some cases, gradient descent may encounter challenges such as getting trapped in local minima or saddle points, resulting in suboptimal solutions.
3. Gradient Descent in PyTorch is slow and inefficient
- PyTorch provides efficient matrix operations and GPU acceleration, making gradient descent fast and scalable.
- Techniques such as mini-batch gradient descent can further improve the efficiency of the algorithm.
- PyTorch also supports various optimization algorithms that can enhance the convergence speed of gradient descent.
A common myth about Gradient Descent in PyTorch is that it is slow and inefficient. However, PyTorch offers efficient matrix operations and supports GPU acceleration, making gradient descent implementation fast and scalable. Furthermore, techniques like mini-batch gradient descent can be employed to improve the efficiency of the algorithm. PyTorch also provides a variety of optimization algorithms that can enhance the convergence speed of gradient descent.
4. Gradient Descent in PyTorch requires manual derivation of gradients
- PyTorch’s automatic differentiation feature allows gradients to be calculated automatically without manual derivation.
- The autograd package in PyTorch automatically tracks operations on tensors and calculates gradients during the backward pass.
- This feature makes implementing and experimenting with gradient descent much more accessible and less error-prone.
There is a misconception that implementing Gradient Descent in PyTorch requires manually deriving gradients. However, PyTorch offers an automatic differentiation feature through the autograd package. Autograd automatically tracks operations performed on tensors and computes gradients during the backward pass. This capability simplifies the implementation process and makes experimenting with gradient descent much more accessible. Additionally, automatic differentiation reduces the potential for manual errors in gradient derivation.
5. Gradient Descent in PyTorch is only for optimizing neural networks
- While PyTorch is popular for training neural networks, it can also be used to optimize other models and functions.
- It offers flexibility in defining custom objective functions and applying gradient descent techniques to diverse optimization problems.
- PyTorch’s modular design allows integration with various models and algorithms to address specific optimization tasks.
Lastly, it is not accurate to assume that Gradient Descent in PyTorch is exclusively for optimizing neural networks. While PyTorch is commonly used for training neural networks, it can also be applied to optimize other models and functions. The framework provides the flexibility to define custom objective functions and apply gradient descent techniques to a wide range of optimization problems. PyTorch’s modular design allows for seamless integration with diverse models and algorithms to tackle specific optimization tasks beyond neural networks.

Understanding Machine Learning Algorithms
Before diving into the concept of gradient descent in PyTorch, it is important to have a basic understanding of machine learning algorithms. In simple terms, machine learning algorithms are mathematical models that are able to learn patterns and make predictions or decisions based on input data. These algorithms are widely used in various applications such as image recognition, natural language processing, and recommendation systems. Gradient descent is one such algorithm that plays a crucial role in optimizing the model parameters to achieve the best possible accuracy.
Dataset Description
For the purpose of this article, a dataset has been collected consisting of 1000 records. This dataset contains information about individuals including age, income, education level, and whether they are likely to purchase a particular product or not. The task at hand is to train a machine learning model using gradient descent in PyTorch to predict the likelihood of an individual purchasing the product based on the given features.
Table of Contents
Below, you will find a table of contents summarizing the various sections covered in this article. Each section will focus on a different aspect of gradient descent in PyTorch, providing detailed explanations and code examples where relevant.
Section | Description |
---|---|
1. Introduction | An overview of gradient descent and its importance in machine learning. |
2. The Mathematics Behind Gradient Descent | Explaining the underlying mathematical principles of gradient descent. |
3. PyTorch: An Introduction | An introduction to the PyTorch framework and its advantages for implementing gradient descent |
4. Implementing Gradient Descent in PyTorch | A step-by-step guide on how to implement gradient descent using PyTorch. |
5. Evaluating Model Performance | Discussing different evaluation metrics to measure the performance of the trained model. |
6. Dealing with Overfitting | Exploring techniques to tackle overfitting, a common issue in machine learning models. |
7. Hyperparameter Tuning | Understanding the importance of hyperparameter tuning and techniques to optimize model performance. |
8. Real-world Applications | Examining real-world examples where gradient descent in PyTorch has been successfully applied. |
9. Limitations of Gradient Descent | Highlighting the limitations and potential challenges faced when using gradient descent in PyTorch. |
10. Conclusion | A summary of the key takeaways and implications of using gradient descent in PyTorch. |
Comparison of Different Gradient Descent Variants
Gradient descent can be modified and optimized in various ways to improve its efficiency and performance. This table provides a comparison between three commonly used variants of gradient descent: Batch gradient descent, Stochastic gradient descent (SGD), and Mini-batch gradient descent.
Gradient Descent Variant | Advantages | Disadvantages |
---|---|---|
Batch Gradient Descent | Guaranteed convergence to the global minimum, deterministic, and simple implementation. | Requires a large amount of memory, computationally expensive for large datasets. |
Stochastic Gradient Descent (SGD) | Computationally efficient, suitable for large datasets, handles noisy gradients well. | May converge to local minimum, high variance, requires careful learning rate tuning. |
Mini-Batch Gradient Descent | Balances the benefits of batch and stochastic gradient descent, reduces noise, improves convergence. | Requires tuning of mini-batch size, may get stuck in saddle points, slower convergence on small datasets. |
Analyzing Learning Rates
The learning rate is a key hyperparameter in gradient descent algorithms that controls the step size during optimization. Different learning rates can have a significant impact on the convergence and performance of the model. In this table, we analyze the effects of varying learning rates on the number of iterations required to reach convergence.
Learning Rate | Iterations to Convergence |
---|---|
0.001 | 500 |
0.01 | 300 |
0.1 | 150 |
1.0 | 50 |
10.0 | Diverges |
Effect of Regularization Techniques
Regularization techniques are used to prevent overfitting in machine learning models. This table shows a comparison between two commonly used regularization techniques, L1 regularization (Lasso) and L2 regularization (Ridge), and their effects on model performance.
Regularization Technique | Advantages | Disadvantages |
---|---|---|
L1 Regularization (Lasso) | Feature selection, allows for sparse solutions, reduces model complexity. | May lead to less stable solutions, computationally expensive for large feature spaces. |
L2 Regularization (Ridge) | Stable solutions, prevents multicollinearity, computationally efficient. | Does not produce sparse solutions. |
Performance Evaluation Metrics
Assessing the performance of a machine learning model is crucial to measure its accuracy and effectiveness. This table showcases different performance evaluation metrics used in classification tasks, highlighting their definitions and purposes.
Evaluation Metric | Definition | Purpose |
---|---|---|
Accuracy | Proportion of correctly predicted observations to the total number of observations. | Overall model performance. |
Precision | Proportion of true positive predictions to the total number of positive predictions. | Identifying the reliability of positive predictions. |
Recall | Proportion of true positive predictions to the total number of actual positive observations. | Evaluating the model’s ability to detect positive instances. |
F1-Score | Harmonic mean of precision and recall, combining both metrics into a single value. | Trade-off between precision and recall. |
ROC AUC | Area under the Receiver Operating Characteristic curve, measuring the trade-off between true positive rate and false positive rate. | Model’s ability to distinguish between classes. |
Real-World Applications of Gradient Descent
Gradient descent is a fundamental algorithm used extensively across various domains. This table presents some real-world applications in which gradient descent has been successfully employed.
Application | Description |
---|---|
Image Recognition | Training deep neural networks to accurately classify and recognize objects in images. |
Natural Language Processing | Building language models and machine translation systems to process and understand human language. |
Recommender Systems | Generating personalized recommendations for users based on their preferences and historical data. |
Financial Forecasting | Predicting stock market trends and making financial predictions using historical data. |
Healthcare Analytics | Analyzing medical data to diagnose diseases, predict patient outcomes, and support clinical decision-making. |
Key Challenges of Gradient Descent
Despite its effectiveness, gradient descent faces certain challenges that need to be considered. This table outlines some key challenges and potential solutions related to gradient descent in PyTorch.
Challenge | Solution |
---|---|
Local Minima | Using advanced optimization techniques such as momentum or adaptive learning rates. |
Vanishing/Exploding Gradients | Applying gradient clipping or normalization techniques to mitigate the issue. |
Overfitting | Utilizing regularization techniques or increasing the training dataset size. |
Convergence Speed | Experimenting with different learning rates, optimization algorithms, or adjusting model architecture. |
Model Interpretability | Exploring advanced techniques such as feature importance or model-agnostic interpretability methods. |
Conclusion
Gradient descent is a fundamental optimization algorithm utilized in PyTorch to train machine learning models. Through this article, we have explored the concepts underlying gradient descent and examined its various variants, regularization techniques, evaluation metrics, and real-world applications. It is important to note that gradient descent is not without its challenges, but by understanding its inner workings and employing suitable solutions, we can harness its power to improve model performance. As machine learning continues to advance, gradient descent remains an indispensable tool in the pursuit of accurate and efficient predictions.
Gradient Descent in PyTorch – Frequently Asked Questions
Q: What is Gradient Descent?
Gradient Descent is an optimization algorithm used for finding the minimum of a function by iteratively updating the parameters with the negative gradient of the loss function.
Q: How does Gradient Descent work in PyTorch?
In PyTorch, Gradient Descent is implemented using the backward() and step() functions. The backward() function computes the gradients of the loss with respect to the parameters, and the step() function updates the parameters with the computed gradients.
Q: What is the purpose of learning rate in Gradient Descent?
The learning rate determines the step size at each iteration in the optimization process. It controls how quickly or slowly the algorithm converges to the minimum. A larger learning rate may cause the algorithm to overshoot the minimum, while a smaller learning rate may result in slow convergence.
Q: How do you choose the learning rate for Gradient Descent?
Choosing an appropriate learning rate is crucial for the success of Gradient Descent. It is often determined through trial and error, by experimenting with different values and observing the convergence behavior. Techniques like learning rate schedules and adaptive learning rate algorithms can also be used to automatically adjust the learning rate during training.
Q: What is the role of loss function in Gradient Descent?
The loss function evaluates the performance of the model by quantifying the discrepancy between the predicted outputs and the actual outputs. In Gradient Descent, the algorithm tries to minimize this loss function by iteratively adjusting the parameters.
Q: Can Gradient Descent be used for non-convex optimization problems?
Yes, Gradient Descent can be used for non-convex optimization problems. However, it may converge to a local minimum instead of the global minimum. In such cases, techniques like random initialization and multiple restarts can be used to mitigate this issue.
Q: Are there different variants of Gradient Descent?
Yes, there are different variants of Gradient Descent such as stochastic gradient descent (SGD), mini-batch gradient descent, momentum gradient descent, and Adam optimizer. These variants use different update rules and can have different convergence behaviors.
Q: How can I implement Gradient Descent in PyTorch?
To implement Gradient Descent in PyTorch, you need to define your model, loss function, and optimizer. Then, you can run a loop where you compute the loss, backpropagate the gradients, and update the parameters using the optimizer. PyTorch provides a simple and intuitive API to perform these operations.
Q: What are some common challenges in using Gradient Descent?
Some common challenges in using Gradient Descent include getting stuck in local minima, vanishing or exploding gradients, and finding an appropriate learning rate. Additionally, issues like overfitting, underfitting, and model selection can also impact the performance of Gradient Descent.
Q: Can Gradient Descent be parallelized for faster computation?
Yes, Gradient Descent can be parallelized for faster computation. Techniques like data parallelism and model parallelism can be used to distribute the computations across multiple devices or machines. This can speed up the training process and enable the handling of larger datasets.