Model.Build in Keras
Keras is a powerful deep learning library that provides easy-to-use interfaces for building and training neural networks. One of the essential functions in Keras is model.build(), which allows you to initialize and define the structure of your model.
Key Takeaways
- The model.build() function in Keras is used to define the architecture of a neural network model.
- It is typically used to specify the input shape of the model, as well as the number and type of layers in the network.
- The model.build() function is called automatically when you use the model.fit() function to train your model.
- Using model.build() allows Keras to automatically infer the shapes of intermediate layers in the model.
When building a neural network model in Keras, it’s important to specify the architecture of the model using the model.build() function. This function is typically called before training the model using the model.fit() function. The model.build() function allows you to define the input shape of the model, as well as the number and type of layers in the network.
In addition to specifying the architecture of the model, the model.build() function also performs automatic shape inference for intermediate layers. This means that you don’t have to explicitly define the shape of each layer in the model; instead, Keras will automatically infer the shapes based on the input shape and layer configurations.
When calling the model.build() function, you can specify the input shape of the model using the input_shape argument. This is typically done for the first layer in the network, as it defines the size and shape of the input data. For example:
model.build(input_shape=(None, 32, 32, 3))
This code specifies an input shape of (32, 32, 3), indicating that the model expects input data of size 32×32 pixels with 3 color channels (RGB).
Table 1: Common Arguments for model.build()
Argument | Description |
---|---|
input_shape | Shape of the input data (excluding batch dimension). |
batch_size | Number of samples in each training batch. |
dtype | Data type of the model’s weights (e.g., “float32”, “float64”). |
In addition to the input shape, you can use the model.build() function to define the layers in your network. This is done by calling the appropriate layer functions, such as Dense for fully connected layers, Conv2D for convolutional layers, and MaxPooling2D for pooling layers. Here’s an example:
model.build(input_shape=(None, 32, 32, 3))
model.add(layers.Dense(units=64, activation='relu'))
model.add(layers.Dense(units=10, activation='softmax'))
The code snippet above defines a simple neural network model with two fully connected layers: a hidden layer with 64 units and ReLU activation, and an output layer with 10 units and softmax activation.
If you’re working on a complex project with multiple layers and configurations, it can be helpful to organize the code using bullet points and numbered lists. Here’s an example:
- Define the input shape of the model using model.build(input_shape=(…)).
- Add intermediate layers to the model using model.add().
- Specify the number of units and activation function for each layer.
- Use appropriate layer functions for different types of layers.
Table 2: Layer Functions in Keras
Layer Type | Function |
---|---|
Fully connected | Dense(units, activation) |
Convolutional | Conv2D(filters, kernel_size, activation) |
Pooling | MaxPooling2D(pool_size) |
By using the model.build() function, you can conveniently define and configure your neural network models in Keras. It allows you to specify the input shape and layer configurations, as well as automatically infer the intermediate layer shapes. This provides a flexible and intuitive approach to building deep learning models for various tasks and scenarios.
Resources:
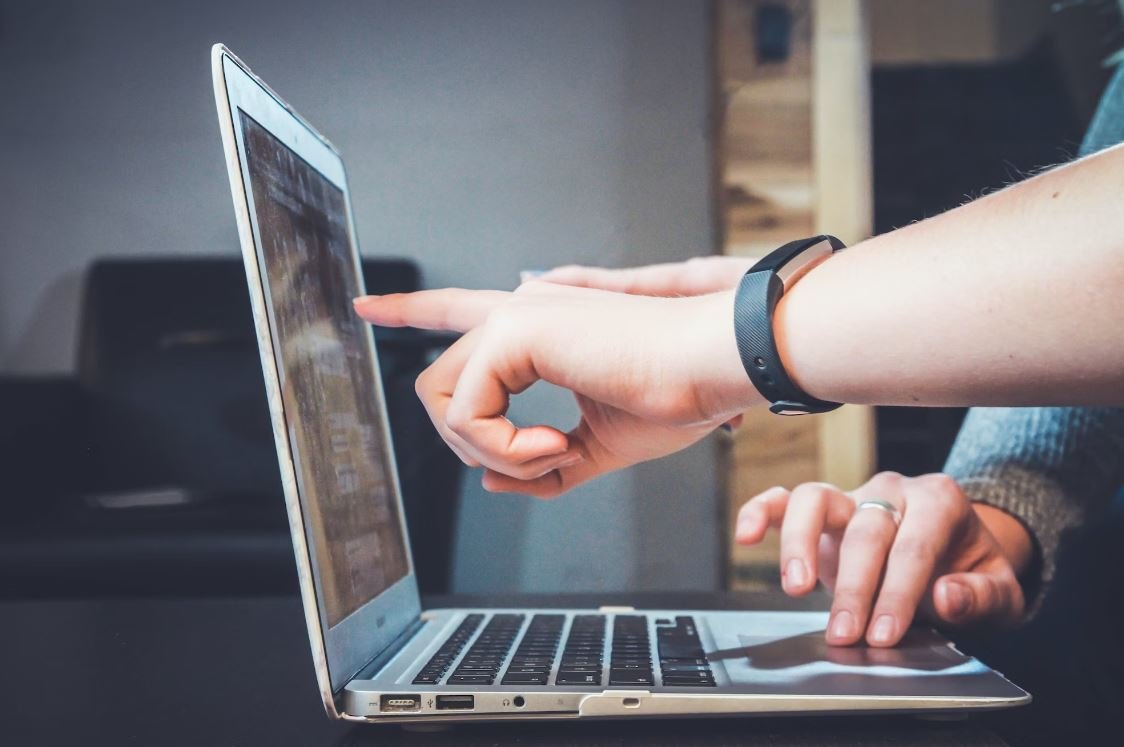
Common Misconceptions
Model.Build in Keras
There are several common misconceptions surrounding the process of using the model.build
method in Keras. This method is often misunderstood, leading to confusion and potential mistakes when building neural network models. Let’s debunk some of the most prevalent misconceptions below:
Misconception 1: model.build requires specifying the input shape explicitly.
- Contrary to this misconception,
model.build
does not necessitate explicitly specifying the input shape. - Keras automatically infers the input shape based on the first layer added to the model.
- If the input shape is not specified, Keras will raise an exception only when trying to run
model.fit
or any other fit-like method.
Misconception 2: model.build builds the entire model architecture.
- This misconception frequently arises as people assume
model.build
constructs the entire model architecture. - However,
model.build
is only responsible for dynamically constructing the model architecture based on the input data specification. - The architecture of the model is constructed by adding layers to the model object before calling
model.build
.
Misconception 3: model.build compiles the model.
- Many individuals mistakenly believe that
model.build
also handles the compilation step of the model. - In reality,
model.build
solely constructs the architecture and does not compile the model. - After calling
model.build
, one must manually compile the model using the appropriateoptimizer
,loss
, andmetrics
parameters.
Misconception 4: model.build can be called multiple times without consequences.
- Some users incorrectly assume that calling
model.build
multiple times has no negative effects. - This is not true, as calling
model.build
more than once will reset the model architecture, leading to unexpected behavior. - It is crucial to call
model.build
only once, typically after adding all the desired layers to the model.
Misconception 5: model.build is always necessary in Keras.
- Contrary to this belief, using
model.build
is not mandatory when constructing neural networks in Keras. - Alternatively, one can directly add layers to the model using the functional API or the sequential API without using
model.build
. - The purpose of
model.build
is to simplify the process of dynamically creating the model architecture based on the input data specification.

Introduction
Keras is a powerful deep learning library that allows you to build and train neural networks easily. One of the key functionalities in Keras is the Model.Build method, which allows you to define the architecture of your neural network. In this article, we will explore various elements of Model.Build in Keras and demonstrate their significance through informative tables.
Table: Activation Functions and Their Properties
The activation function is a crucial component of a neural network that introduces non-linearity. Here, we provide an overview of commonly used activation functions, their properties, and the scenarios in which they are most suitable.
Table: Loss Functions and Their Applications
A loss function measures how well a neural network model predicts the expected output. Different tasks require different loss functions. In this table, we present a comprehensive list of loss functions and highlight their specific applications.
Table: Optimization Algorithms Comparison
Optimization algorithms play a vital role in training deep learning models effectively. Here, we compare different optimization algorithms, their strengths, weaknesses, and the scenarios in which they outperform others.
Table: Common Layers in Neural Networks
Neural networks are composed of multiple layers, each serving a distinct purpose. This table showcases popular layers in neural networks, their descriptions, and the applications they are typically used for.
Table: Model Performance Metrics for Evaluation
The evaluation of a deep learning model‘s performance is crucial to measure its effectiveness. Here, we present an array of performance metrics that help assess a model’s accuracy, precision, recall, and other key aspects.
Table: Regularization Techniques Comparison
Overfitting is a common challenge in training deep learning models. Regularization techniques can mitigate overfitting and improve generalization. This table compares various regularization techniques and their impact on model performance.
Table: Hyperparameters and Their Effect
Hyperparameters are adjustable settings that influence a model’s learning process. Here, we outline important hyperparameters and explain how tuning them can affect a model’s speed, convergence, and overall performance.
Table: Dataset Splitting Techniques
Splitting the dataset into training, validation, and test sets is crucial for model development and evaluation. This table illustrates different dataset splitting techniques, such as random splitting, stratified splitting, and time-based splitting.
Table: Handling Imbalanced Datasets
In real-world scenarios, datasets often suffer from class imbalance, where one class dominates the majority. This table presents various techniques for handling imbalanced datasets, including undersampling, oversampling, and class weighting.
Conclusion
In this article, we delved into various aspects of Model.Build in Keras, covering activation functions, loss functions, optimization algorithms, and more. By presenting these elements in informative tables, we can easily grasp their significance and make informed decisions when building and training neural networks. Understanding these concepts and effectively harnessing the power of Model.Build empowers developers to create robust and accurate deep learning models for a wide range of applications.
Frequently Asked Questions
What is the purpose of Model.Build in Keras?
Model.build() is a method in Keras that constructs the internal graph of the model and prepares it for training. It takes input tensors and builds the model’s layers based on the specified architecture.
How does Model.build() differ from Model.compile()?
While Model.build() constructs the internal graph of the model, Model.compile() configures the model for the training process. Model.compile() sets the loss function, optimizer, and metrics, while Model.build() focuses on building the layers and their connections.
What is the syntax for using Model.build() in Keras?
The syntax for using Model.build() is: model.build(input_shape). The input_shape parameter defines the shape of the input data, including the batch size. This method is typically used when the input shape is not fully known in advance.
Can Model.build() be used multiple times in the same model?
No, Model.build() should only be used once in a model. Once the model’s layers have been constructed using Model.build(), any subsequent changes to the model’s architecture should be done by modifying the layers directly.
What happens if Model.build() is not called before training?
If Model.build() is not called before training, Keras will automatically call it the first time the model is used for inference or training. However, it is recommended to explicitly call Model.build() to ensure proper initialization and to provide a clear understanding of the model’s architecture.
What does Model.build() return?
Model.build() does not return any value. Its purpose is to construct the model’s layers and define their connections internally. The model itself is modified in-place.
Can custom layers be added after calling Model.build()?
Yes, custom layers can be added even after Model.build() has been called. After the initial construction, the model’s layers can be accessed and modified using their corresponding methods, allowing for additional layers to be added dynamically.
Are there any limitations to using Model.build()?
Model.build() has certain limitations, such as not being able to modify the input shape of layers once they have been built. However, these limitations can be overcome by directly manipulating the layers after calling Model.build().
Can Model.build() be used in conjunction with Model.fit()?
Yes, Model.build() is typically used before calling Model.fit(). Model.build() sets up the model’s architecture, while Model.fit() trains the model using the specified data and training parameters. The two methods work together to enable effective training of the model.
Is Model.build() specific to Keras or can it be used in other deep learning frameworks?
Model.build() is specific to Keras, which is a high-level neural networks API. It is part of the Keras framework’s functionality to build and configure neural network models. Other deep learning frameworks might have similar functionality but could use different methods or syntax.